Key Construction Decisions
Chapter 4
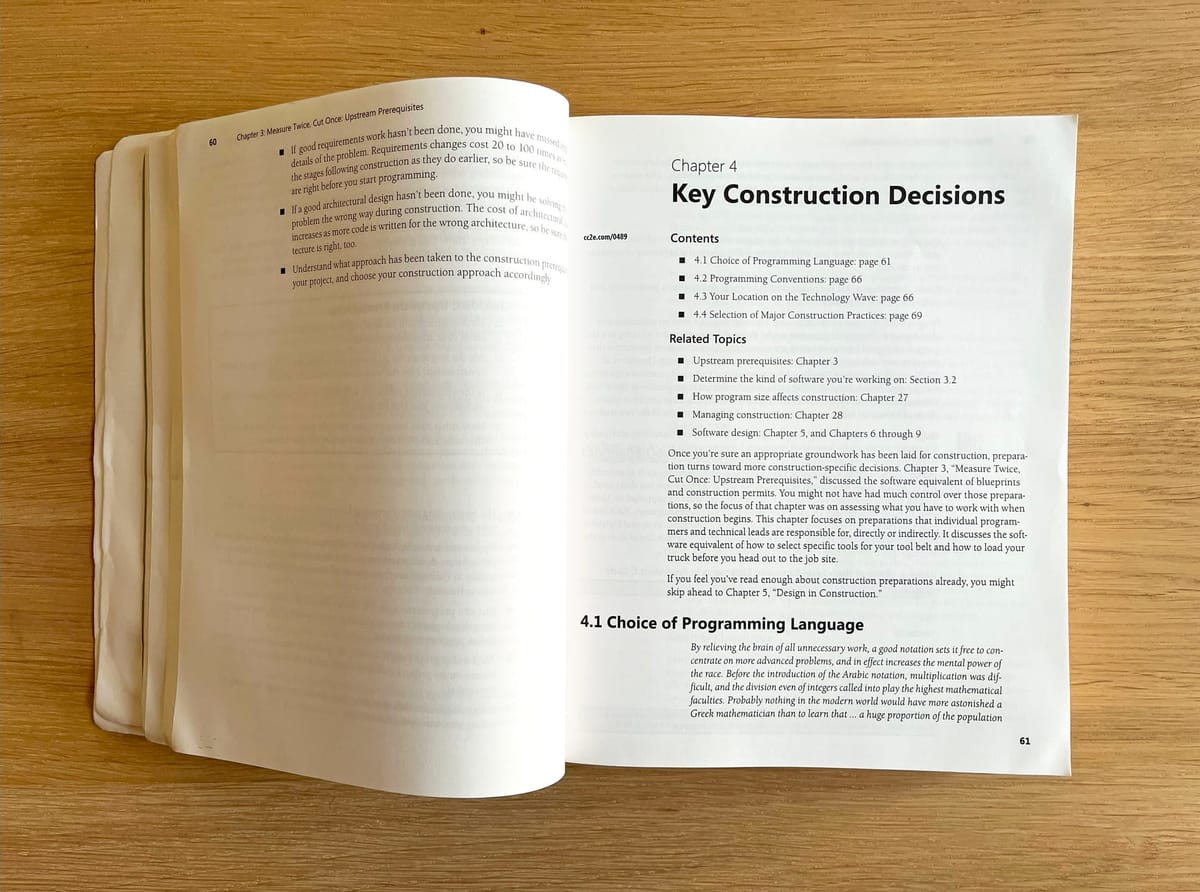
This week is called key construction decisions, but its really about languages—programming languages and the conventions associated with each particular way of writing code. Choosing a language to write in is not often a choice that people have the opportunity to make. Most people have one native tongue and perhaps have passing knowledge of one or two other languages (three if they're lucky). If they're an English-speaking American, it's likely that they don't know any other languages at all. When writing a complex technical document, there's often just one choice no matter how many languages you can speak. When writing code, on the other hand, there are often many possible languages to choose from. Since the 1940s, hundreds of programming languages have been invented, and it's not uncommon for experienced software engineers to be "fluent" in many common-purpose languages and perhaps several esoteric languages for specific use cases.
The choice of which language to use for any given project has as much to do with the programmer's familiarity as it does for its designed purpose. Familiarity begets quality and productivity, whereas the language itself offers varying levels of abstraction and automation that are necessary to translate the program's complex requirements into machine-executable code. In this chapter, McConnell references an interesting idea of linguistic relativity called the Sapir-Whorf hypothesis—the idea that our ability to have certain thoughts is dependent on knowing the language to describe those thoughts. In the context of computer code, the words and structures available in various languages both hinder and influence our ability to express ideas in a way that is functional and relevant to the desired output. I found this discussion of the Sapir-Whorf hypothesis fascinating, and further reading suggested compelling arguments both for and against its relevance to linguistic reality. The implications of this hypothesis are far-reaching and could be used as a lens for investigating all sorts of expression, including architectural design, music, and literature.
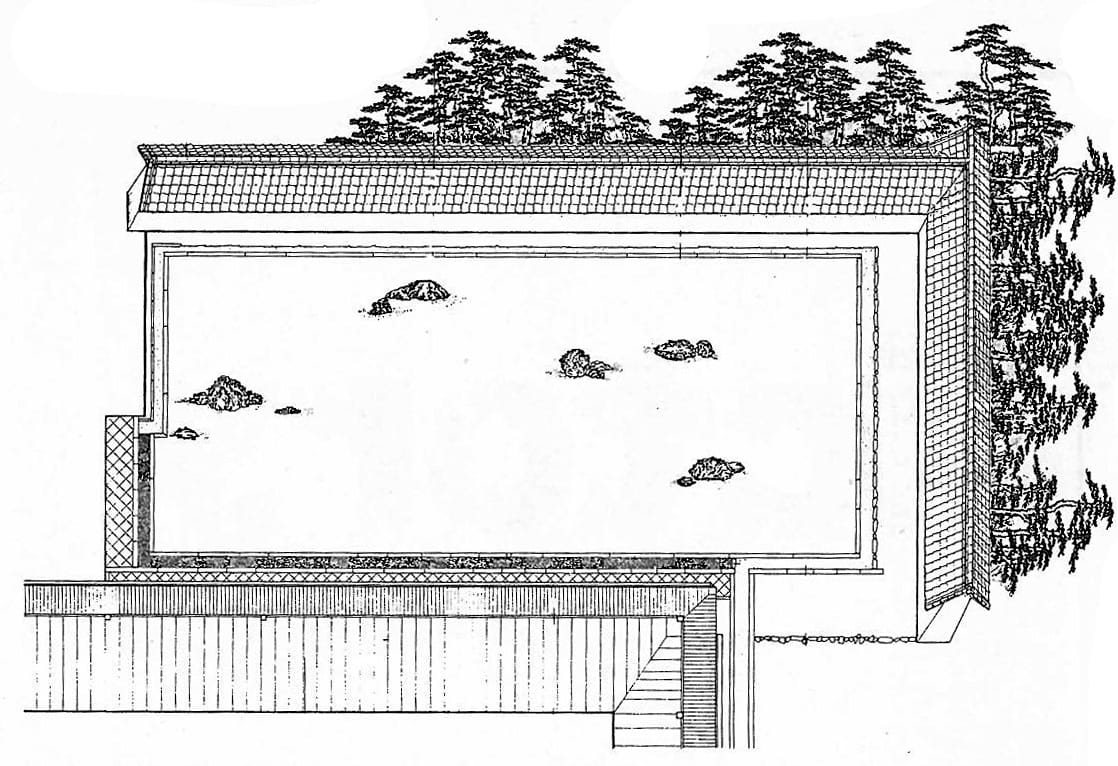
Side Quest: further information about "linguistic relativity"
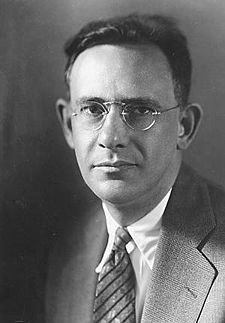


Video: Professor Robert Sapolsky from Stanford explains linguistic relativity (link)
McConnell uses this hypothesis to illustrate a phenomenon he's observed in the programming world, in which people tend to bring their old habits with them as they switch from one language to another. Old bad habits can manifest in new languages either as inefficiencies in the code itself or in people's inability to grasp the opportunities afforded to them by a new programming paradigm.
When embarking on a new project, the choice of language is important because it's a crucial moment when you have to balance the abilities and understanding of the workers with the potential technical benefits of the language. An understanding of your collaborators' experience and fluency is just as important as comparative knowledge of what your choices are in terms of tools to use. It's possible that an imperfect or outdated tool—when used with skill and care—can far out-perform the latest technology in the hands of a neophyte.
Despite the existence of hundreds of programming languages in the broad, abstract world of computer science, there are industry-specific or application-specific constraints that often dramatically limit the possible practical choices. As this is a blog for architecture and engineering professionals who are interested in computer science and programming, the AEC sphere is the constraint in question for our present investigation of different programming languages. This chart lists a few (certainly not all) popular programs used in architecture and engineering for design, documentation, and visualization:
Software Name | Core Language | Application Programming Interface (API) Languages |
---|---|---|
Rhino | C++ | C++, .NET (C#), Python, RhinoScript*, Grasshopper* |
Revit | C++ | .NET (C#), Python, Dynamo* |
SketchUp | C++ | C, Ruby |
ArchiCAD | C++ | C++, Python, GDL* |
AutoCAD | C++ | C++, .NET (C#), AutoLISP* |
Civil 3D | C++ | C++, .NET (C#), AutoLISP* |
MicroStation | C++ | C++, .NET (C#), VBA, MDL* |
Vectorworks | C++ | Python, VectorScript*, Marionette* |
Blender | C, C++ | Python |
Unreal Engine | C++ | C++, Python, Blueprints* |
Maya | C++ | C++, Python, MEL* |
SolidWorks | C++ | C++, .NET (C#), VBA |
Catia | C++ | C++, .NET (C#), VBA |
Tekla Structures | C++ | .NET (C#) |
*Proprietary software-specific programming interfaces/scripting languages
Without knowing anything at all about programming languages, you might infer a couple things from this list: A) All software that handles geometric data seems to come from a common programming language called C++; and B) the most common ways to interact programmatically with these programs (aside from the core language) are through two languages called ".NET (C#)" and "Python." If you don't in fact know anything about programming languages, check out this quick primer, otherwise skip ahead:
AEC Programming Languages In a Nutshell
-
Core Language
-
C++ is a statically-typed, compiled, low-level, general-purpose programming language first released in 1985 as a successor to C. It's often used in resource-intensive applications where careful management of the computer's memory and performance are required. This is why we find it at the heart of all AEC software, where vast amounts of geometric data must be parsed in realtime. C++ has a very difficult learning curve; one can easily introduce bugs that will crash the computer. In the words of its creator Bjarne Stroustrup: "C makes it easy to shoot yourself in the foot; C++ makes it harder, but when you do it blows your whole leg off." For that reason, it is preferable to use safer languages when interacting with an API. C++ compiles directly to machine-readable binary that is specific to whatever hardware is running it.
-
API Languages
- C# is a mostly-statically-typed, compiled, high-level, general purpose programming language first released in 2000. It features automations that make the language safer to use, at a slight cost of performance in resource-intensive operations. It's the primary (but not the only) language used in the .NET open-source development framework published by Microsoft. The extensibility of C# through the .NET framework is a powerful feature that allows AEC softwares to interact with other external libraries or each other. C# compiles to an intermediate language that is itself compiled during program execution to machine-readable binary by the .NET runtime. This allows for the same compiled code to be run in different environments.
- Python is an dynamically-typed, interpreted, high-level, general-purpose programming language first released in 1991. The principle design goal of Python was to create a language that was easily read by humans. Instead of using various characters to separate logic within a program, Python uses line breaks and indentation (similar to how people write paragraphs or dialogue). For this reason it's considered to be a good first language to learn and is the point of entry to coding for many people. Because Python is an interpreted language (i.e. the source code is read by the computer at the moment it's executed), it must be run through the use of an installed interpreter. This imposes a significant penalty on performance in exchange for user-friendliness. The Python interpreter is itself written in C, but there is a variant interpreter written in C# that allows for Python programs to access .NET resources and libraries. This flavor of Python is called IronPython, and it's used in several AEC softwares in place of (or alongside) the more commonly-used CPython. It is important for AEC programmers to understand that IronPython is deprecated and is not compatible with more current versions of CPython.
Given that C# and Python have the most overlap when using AEC APIs to develop automations and plugins, it's common for computational designers and BIM specialists to develop computational thinking through visual programming interfaces like Grasshopper or Dynamo, start learning how to code in Python due to its ease of use, and later switch to C# when greater control and complexity is needed. Even though the scope of choices available to programmers in this space is so limited, McConnell's assertion of the Sapir-Whorf hypothesis still holds weight, as these options are so different from one another. It has been my experience that familiarity with one way of programming can hinder or slow our development in other areas and cause us to see every problem through the lens of our favored interface. The primary impetus for this blog was the desire to break out of this way of thinking and learn to approach computational problems from different angles.
Programming Into a Language
One method for achieving greater flexibility is to program into our chosen language as opposed to programming in it. When we write code in Python or write code in C#, we allow the strictures of each specific language dictate to us what is or is not appropriate or feasible. When programming into a language, we first decide in abstract terms what we want to express and how we want to do it, then we figure out how to get it done with the tools available.
Most of the important programming principles depend not on specific languages but on the way you use them. If your language lacks constructs that you want to use or is prone to other kinds of problems, try to compensate for them. Invent your own coding conventions, standards, class libraries, and other augmentations.
Programming Conventions
Another way we can try to push back against this sort of tunnel vision is to plan ahead and define clear programming conventions before we start writing code. Last week we reviewed the main goals for software architecture. Essentially, the architecture of a program (its high-level design) is intended to ensure conceptual integrity between the requirements of a program and its implementation (its detailed design). Programming conventions should provide for a clear and unhindered relationship between the architecture and the construction of a project.
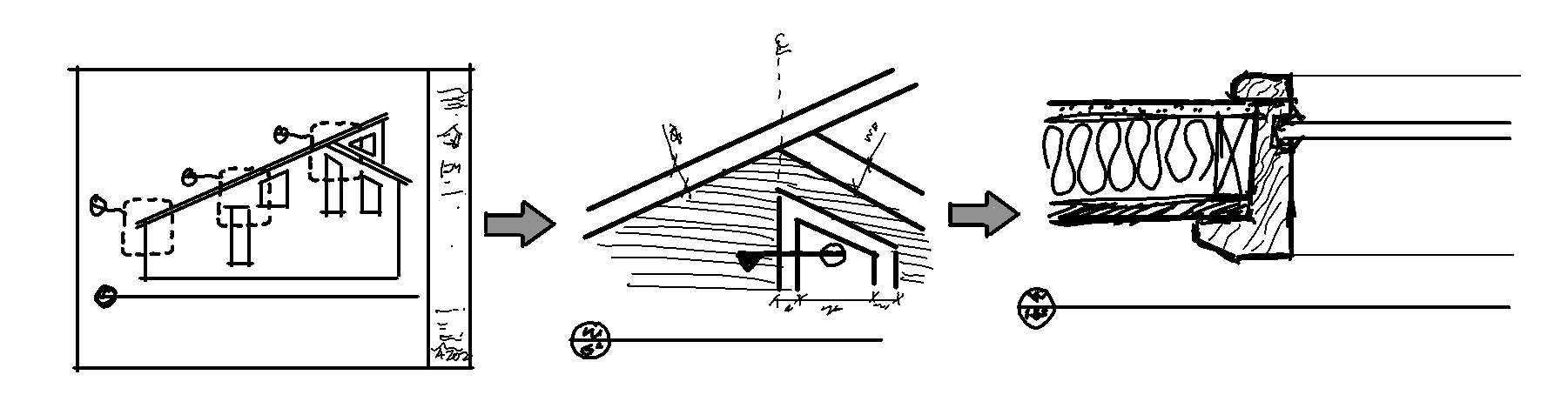
In architectural drawing sets, larger-scale drawings (such as the elevation of an entire building) call out detailed areas (such as a specific window type) that require special attention of the builder, due to special design considerations or a particularly complex assembly. Clear and orderly drawing standards allow for an unhindered flow of information from the architectural guidelines to the construction guidelines. Programming conventions are akin to drawing standards—an effort to impose a unifying discipline on the detailed design of a project. This helps to avoid arbitrary style differences that make it difficult for the brain to parse detailed information while simultaneously holding onto the broader context.
Before you start coding you must decide on programming conventions, as this quickly becomes nearly impossible to change later on. Readers employed in architectural practice will most likely have dark memories of being on one or two projects that lost their way, ending up with a messy and incomprehensible BIM model whose maintenance consumes countless hours of confusion and wasted labor. As a computational design specialist employed at one such architecture firm, I preach standardization as if it were gospel—and... sometimes it's heard. The idea of programming conventions in software development comes to me quite naturally, and I can indeed appreciate how difficult it is to insert standardization into an existing unordered system.
Technology Waves
Another important thing to consider before writing any code is the project's position on whatever technology wave it's riding. Technologies come and go—some are just a flash in the pan while others persist for decades or centuries—but there is a clear cycle of relevance for technology (certainly for all digital technology). Any software project will invariably rest on some point on some tech wave, and it's important to acknowledge that point before you begin. If you happen to find yourself comfortably situated at the crest of a wave, you can enjoy broad support and bountiful expertise in your field. The tools you use will be bug-free, fully-developed, and largely integrated with one another. If, on the other hand, you find yourself a pioneer in some as-yet-unproven field of digital technology, things will be very different. Your choice of languages will be limited, and the tools available to you will primitive or not fully-developed yet. Expertise will have to be earned, as you might be the first person ever to encounter whatever problems you face. Significant amounts of time will have to be budgeted for creative problem-solving instead of production, but with success comes the value in creating something completely new.
This is not a recommendation for seeking out either path; your point on the wave is often totally out of your control due to any number of variables. Being aware of that point right at the beginning is important, though, as it can suggest certain conventions that might prove invaluable in the long run. For example, a project situated in an early-wave environment might benefit greatly from an explicitly-defined policy of programming into the available tools to achieve some of the benefits of a more mature technology environment.
Ultimately, the choice of language and convention has as much to do with personal preference as it does with any of the reasons discussed here. If it makes you happy doing things a certain way, you should probably follow your instincts. Among my computational design colleagues, there is some good-natured quarreling over the comparative merits of Python and C#. We're fortunate, perhaps, that the whims of capital and industry have limited our choices to such an extent—and that their differences usually make the choice pretty easy for most people. The true crisis of confidence comes later, when computational designers venture out from their design software APIs and onto the web, where it seems as if technological waves oscillate and crash on a weekly basis.
Comments ()